Rust是一種強(qiáng)類型、高性能的系統(tǒng)編程語言,其官方文檔中強(qiáng)調(diào)了Rust的標(biāo)準(zhǔn)庫具有良好的并發(fā)編程支持。Thread是Rust中的一種并發(fā)編程方式,本文將介紹Rust中thread的相關(guān)概念、方法和字段、常見用法以及多線程的一些實(shí)踐經(jīng)驗(yàn)。由淺入深帶你零基礎(chǔ)玩轉(zhuǎn)Rust的多線程編程。
線程的基本概念和使用方法
Thread是Rust中并發(fā)編程的一種基本方式。Rust中的Thread使用標(biāo)準(zhǔn)庫中的std::thread::Thread
結(jié)構(gòu)體表示。我們可以通過下面的代碼來創(chuàng)建一個(gè)Thread:
use std::thread;
fn main() {
let handle = thread::spawn(|| {
// 子線程執(zhí)行的代碼
});
}
其中的||
表示閉包,該閉包中的代碼將在子線程中執(zhí)行。調(diào)用thread::spawn
方法會(huì)返回一個(gè)Result,該Result包含一個(gè)智能指針,該智能指針擁有對(duì)線程的所有權(quán),如果線程執(zhí)行成功則返回Ok,否則返回Err。通過這個(gè)智能指針我們可以管理線程的生命周期和操作線程。
當(dāng)線程中的代碼執(zhí)行完畢時(shí),我們可以使用以下代碼將線程加入主線程:
handle.join().expect("執(zhí)行失敗");
Thread也支持通過std::thread::Builder
結(jié)構(gòu)體進(jìn)行創(chuàng)建,Builder提供了一些線程的配置項(xiàng),如線程名字、線程優(yōu)先級(jí)、棧大小等。
use std::thread;
fn main() {
let builder = thread::Builder::new().name("my_thread".into());
let handle = builder.spawn(|| {
// 子線程執(zhí)行的代碼
});
}
線程的字段和方法
Thread結(jié)構(gòu)體中提供了一些有用的字段和方法。
線程名稱
Rust中的Thread對(duì)象有一個(gè)名稱屬性,可以通過thread::current()
函數(shù)獲取當(dāng)前線程的名稱,也可以通過std::thread::Builder
結(jié)構(gòu)體設(shè)置線程的名稱。
use std::thread;
fn main() {
let thr0 = thread::current();
let thread_name = thr0.name().unwrap_or("unknown");
println!("當(dāng)前線程的名稱:{}", thread_name);
let builder = thread::Builder::new().name("my_thread".into());
let handle = builder.spawn(move || {
let thr = thread::current();
let name = thr.name().unwrap_or("unknown");
println!("當(dāng)前線程的名稱:{}", name);
});
handle.expect("執(zhí)行失敗").join().unwrap();
}
// 輸出結(jié)果:
// 當(dāng)前線程的名稱:main
// 當(dāng)前線程的名稱:my_thread
線程id
Rust中的Thread對(duì)象還有一個(gè)id屬性,可以通過thread::current()
函數(shù)獲取當(dāng)前線程的id,也可以通過std::thread::Builder
結(jié)構(gòu)體設(shè)置線程的id。
use std::thread;
fn main() {
let thread_id = thread::current().id();
println!("當(dāng)前線程的id:{:?}", thread_id);
let builder = thread::Builder::new().name("my_thread".into());
let handle = builder.spawn(|| {
let id = thread::current().id();
println!("當(dāng)前線程的id:{:?}", id);
});
handle.expect("執(zhí)行失敗").join().unwrap();
}
// 輸出結(jié)果:
// 當(dāng)前線程的id:ThreadId(1)
// 當(dāng)前線程的id:ThreadId(2)
線程休眠
Rust中Thread對(duì)象提供了一個(gè)sleep方法,用于讓線程休眠指定時(shí)間。
use std::{thread, time};
fn main() {
println!("線程休眠前:{:?}", time::Instant::now());
thread::sleep(time::Duration::from_secs(2));
println!("線程休眠后:{:?}", time::Instant::now());
}
// 輸出結(jié)果:
// 線程休眠前:Instant { tv_sec: 9667960, tv_nsec: 471430161 }
// 線程休眠后:Instant { tv_sec: 9667962, tv_nsec: 471515229 }
線程狀態(tài)
Rust中Thread對(duì)象表示的是系統(tǒng)中的一個(gè)線程,可以通過thread::JoinHandle結(jié)構(gòu)體的is_finalized()和thread::Thread的panicking()方法來查看線程是否結(jié)束和是否因panic而結(jié)束。
use std::thread;
fn main() {
let handle = thread::spawn(|| {
// TODO: 執(zhí)行耗費(fèi)時(shí)間的任務(wù)
});
while !handle.is_finished() {
thread::sleep_ms(100);
}
if thread::panicking() {
println!("線程因panic而結(jié)束");
} else {
println!("線程正常結(jié)束");
}
}
常用用法和示例
單線程執(zhí)行
我們可以使用Thread開啟一個(gè)單線程,并在該線程中執(zhí)行我們的代碼。當(dāng)該線程執(zhí)行完畢后,我們通過JoinHandle.join()方法將該線程加入主線程。
use std::thread;
fn main() {
let handle = thread::spawn(|| {
println!("Hello Thread!");
});
handle.join().unwrap();
}
多線程執(zhí)行
我們可以使用多個(gè)Thread對(duì)象并行地執(zhí)行任務(wù),實(shí)現(xiàn)多線程編程。
use std::thread;
fn main() {
let handle1 = thread::spawn(|| {
for i in 0..5 {
println!("Thread1: {}", i);
}
});
let handle2 = thread::spawn(|| {
for i in 0..5 {
println!("Thread2: {}", i);
}
});
handle1.join().unwrap();
handle2.join().unwrap();
}
線程間通信
Rust中線程間通信可以通過channel實(shí)現(xiàn)。在以下例子中,我們開啟兩個(gè)線程,一個(gè)線程向channel發(fā)送數(shù)據(jù),另一個(gè)線程從channel接收數(shù)據(jù)。兩個(gè)線程可以通過channel實(shí)現(xiàn)數(shù)據(jù)共享和交換。
use std::thread;
use std::sync::mpsc;
fn main() {
let (tx, rx) = mpsc::channel();
let handle1 = thread::spawn(move || {
tx.send("Hello Thread!".to_string()).unwrap();
});
let handle2 = thread::spawn(move || {
let msg = rx.recv().unwrap();
println!("{}", msg);
});
handle1.join().unwrap();
handle2.join().unwrap();
}
進(jìn)階用法:多線程協(xié)作和鎖
多線程協(xié)作
當(dāng)線程之間需要協(xié)作執(zhí)行任務(wù)時(shí),我們可以通過Rust中提供的互斥鎖Mutex和讀寫鎖RwLock來實(shí)現(xiàn)。
以下是一個(gè)簡(jiǎn)單的例子,在這個(gè)例子中我們開啟兩個(gè)線程,一個(gè)線程向共享變量加1,另一個(gè)線程向共享變量減1。由于有兩個(gè)線程同時(shí)修改共享變量,我們需要使用Mutex來進(jìn)行加鎖和解鎖操作。
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
let shared_count = Arc::new(Mutex::new(0));
let thread1 = shared_count.clone();
let handle1 = thread::spawn(move || {
for _ in 0..10 {
let mut count = thread1.lock().unwrap();
*count += 1;
}
});
let thread2 = shared_count.clone();
let handle2 = thread::spawn(move || {
for _ in 0..10 {
let mut count = thread2.lock().unwrap();
*count -= 1;
}
});
handle1.join().unwrap();
handle2.join().unwrap();
println!("shared_count: {:?}", *shared_count.lock().unwrap());
}
// 輸出結(jié)果:
// shared_count: 0
鎖
在多線程編程中,鎖是一種常見的同步機(jī)制,它用于保護(hù)共享數(shù)據(jù)不受到并發(fā)訪問的影響。Rust標(biāo)準(zhǔn)庫中提供了鎖的實(shí)現(xiàn)Mutex、RwLock、Barrier、Condvar等等。
Mutex
Mutex是Rust中最基本的鎖機(jī)制,它提供了互斥訪問的機(jī)制。當(dāng)多個(gè)線程同時(shí)對(duì)一個(gè)共享資源進(jìn)行訪問時(shí),Mutex會(huì)對(duì)該資源進(jìn)行加鎖,當(dāng)一個(gè)線程訪問該資源時(shí),其他線程無法訪問該資源,直到該線程解鎖該資源。
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
let shared_data = Arc::new(Mutex::new(0));
let thread1 = shared_data.clone();
let handle1 = thread::spawn(move || {
for _ in 0..10 {
let mut data = thread1.lock().unwrap();
*data += 1;
}
});
let thread2 = shared_data.clone();
let handle2 = thread::spawn(move || {
for _ in 0..10 {
let mut data = thread2.lock().unwrap();
*data -= 1;
}
});
handle1.join().unwrap();
handle2.join().unwrap();
println!("shared_data: {:?}", *shared_data.lock().unwrap());
}
// 輸出結(jié)果:
// shared_data: 0
RwLock
RwLock是一種讀寫鎖,它提供了兩種訪問方式:讀取訪問和寫入訪問,當(dāng)同時(shí)有多個(gè)讀操作時(shí),RwLock會(huì)共享鎖,允許多個(gè)線程同時(shí)訪問該數(shù)據(jù),當(dāng)進(jìn)行寫操作時(shí),RwLock會(huì)對(duì)該數(shù)據(jù)進(jìn)行排它鎖,只允許一個(gè)線程進(jìn)行訪問。
use std::sync::{Arc, RwLock};
use std::thread;
fn main() {
let shared_data = Arc::new(RwLock::new(0));
let thread1 = shared_data.clone();
let handle1 = thread::spawn(move || {
for _ in 0..10 {
let mut data = thread1.write().unwrap();
*data += 1;
}
});
let thread2 = shared_data.clone();
let handle2 = thread::spawn(move || {
for _ in 0..10 {
let data = thread2.read().unwrap();
println!("data: {:?}", *data);
}
});
handle1.join().unwrap();
handle2.join().unwrap();
println!("shared_data: {:?}", *shared_data.read().unwrap());
}
// 輸出結(jié)果:
// data: 10
// data: 10
// data: 10
// data: 10
// data: 10
// data: 10
// data: 10
// data: 10
// data: 10
// data: 10
// shared_data: 10
RwLock還提供了一個(gè)try_read()方法,可以進(jìn)行非阻塞式的讀操作。
Barrier
Barrier是一種同步機(jī)制,它提供了一個(gè)點(diǎn),當(dāng)多個(gè)線程只有在該點(diǎn)處到達(dá)才能繼續(xù)執(zhí)行。Barrier有一個(gè)計(jì)數(shù)器,當(dāng)計(jì)數(shù)器到達(dá)值N時(shí),所有在該Barrier處等待的線程可以繼續(xù)執(zhí)行。
use std::sync::{Arc, Barrier};
use std::thread;
fn main() {
let barrier = Arc::new(Barrier::new(3));
let thread1 = barrier.clone();
let handle1 = thread::spawn(move || {
println!("Thread1 step1.");
thread1.wait();
println!("Thread1 step2.");
});
let thread2 = barrier.clone();
let handle2 = thread::spawn(move || {
println!("Thread2 step1.");
thread2.wait();
println!("Thread2 step2.");
});
handle1.join().unwrap();
handle2.join().unwrap();
}
// 輸出結(jié)果:
// Thread1 step1.
// Thread2 step1.
// ERROR Timeout
最佳實(shí)踐:安全地使用Thread
在使用Thread進(jìn)行多線程編程時(shí),為了保證線程安全,我們需要注意以下幾點(diǎn):
- ? 在多線程程序中避免使用靜態(tài)變量,單例模式和全局變量,這些變量可能被多個(gè)線程同時(shí)訪問。
- ? 在多線程編程中,一定要避免使用裸指針和內(nèi)存共享,這種方式可能導(dǎo)致數(shù)據(jù)競(jìng)爭(zhēng)和未定義行為。
- ? 使用Rust的鎖機(jī)制Mutex和RwLock等,保證共享數(shù)據(jù)的線程安全性。
- ? 編寫多線程程序時(shí),應(yīng)該考慮線程池的設(shè)計(jì),防止創(chuàng)建過多的線程帶來的資源錯(cuò)亂和性能損失。
- ? 多線程程序的并發(fā)度一定要注意控制,過高的并發(fā)度反而會(huì)導(dǎo)致性能下降。
以上都是在使用Thread時(shí)應(yīng)該注意的一些安全問題,遵循這些原則可以提高多線程程序的可維護(hù)性和安全性。
總結(jié)
本章節(jié)通過代碼示例深入的探討了Rust中thread的線程的基本概念,線程的字段和方法,常用用法和示例,多線程協(xié)作和鎖以及thread最佳實(shí)踐經(jīng)驗(yàn)。
-
代碼
+關(guān)注
關(guān)注
30文章
4779瀏覽量
68521 -
多線程編程
+關(guān)注
關(guān)注
0文章
17瀏覽量
6687 -
Thread
+關(guān)注
關(guān)注
2文章
83瀏覽量
25923 -
Rust
+關(guān)注
關(guān)注
1文章
228瀏覽量
6599
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
Python多線程編程原理
多線程解決思路一
嵌入式Linux多線程編程
QNX環(huán)境下多線程編程
linux多線程編程開發(fā)
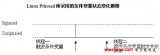
關(guān)于多線程編程教程及經(jīng)典應(yīng)用案例的匯總分析
linux多線程編程技術(shù)
什么是多線程編程?多線程編程基礎(chǔ)知識(shí)
多線程編程指南的PDF電子書免費(fèi)下載
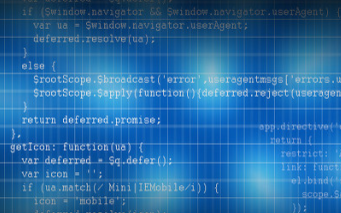
評(píng)論